Pagination is simply the process of segregating information into separate pages as we see in books or reports. We are used to pagination in our everyday life and work rather heavily but often make very little notice of it. In terms of digital content, pagination refers to dividing the contents, words, images or videos, in a website or an app into individual pages.
What is Pagination in Web or Mobile Apps?
Just like in books, pagination helps users to navigate across pages and locate or fetch their desired data online very quickly. We very commonly across pagination in the results pages of search engines, product lists of eCommerce sites and apps, and in our email boxes. Needless to say, these are merely the commonest examples that we actively see and use, pagination is applied to a much more widespread extent across various web contents. It subtly increases the user-friendliness of your website or apps several folds without needing a lot of effort from the developers’ end.
A Large Amount of Data at a Small Amount of Headache
It is important to note at this point that pagination is not merely meant for making things easy for the users. Any web application that needs to fetch a large amount of data and render it on the users’ screens is prone to slow down considerably. Web applications render data on user screens by gathering them from servers through APIs. A large amount of data invariably means a large amount of DOM elements on the webpage that your web application has to process on the front-end. There are a few ways we can use to overcome this problem and maintain high scalability for our web applications.
Progressive loading features are commonly used that give apps the feature of infinite virtual scrolling. In this way, data is progressively loaded by the web apps as the user keeps scrolling through a webpage. Ever feel like you have been scrolling through your social media feed till your thumbs are sore, but never reach the end of your feed? That is a prime example of the infinite virtual scrolling feature. It is most commonly seen in social media apps and some eCommerce apps where it is not important for the user to locate specific items on the webpage, but only view the contents present.
Pagination is more useful when you need to present your content in a more organised form to your users.
Creating Pagination in ReactJS
As an app developer, it is rather useful to have the knowledge of creating pagination in your arsenal. In this blog, we would discuss how to create pagination for your apps or webpages in ReactJS. ReactJS is the most popular JS library for developing user interfaces, mainly for its super extensive size.
Using the In-Built Pagination Libraries in ReactJS
Creating pagination using ReactJS is quite easy. There are already a number of in-build pagination libraries present in ReactJS, like react-js-pagination, react-paginate, and react-bootstrap/Pagination so there is no need for us to reinvent the wheel here.
Let us see here briefly how to use the react-js-pagination component to create pagination for our app.
First, we consider the props. Let us say the total number of pages in our app is 50 and the total number of items is 1500. So we have to include 30 items per page. Let us say we want to display a range of 5 pages at the pagination bar at a time.
Next, we can install the react-js-pagination component with npm using the command:
Now we can simply fill in the props and complete the code for our app in JS as follows:
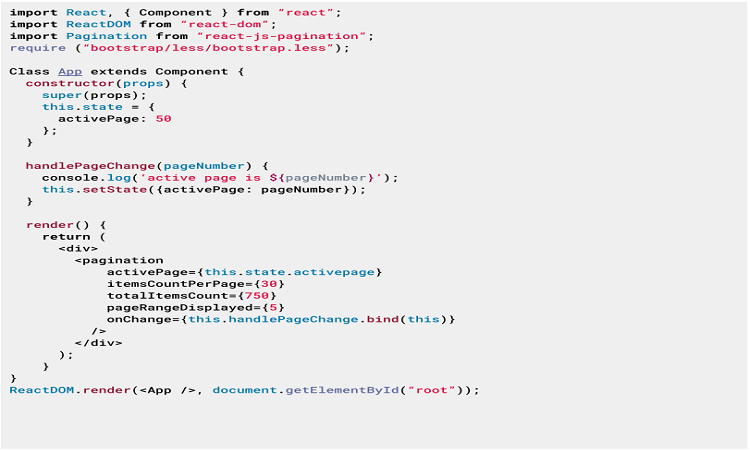
We can clearly see here that there is no room for much customisation in this component. The in-built libraries usually suffer from such a lack of customisations but these are undoubtedly extremely helpful to quickly add pagination to your apps.
What If We Need Customisations Though?
Customisations are always highly sought in Reactjs web development. They allow us the freedom to use a complete range of our imaginations in the apps we build. This in turn makes our apps unique, enhances UI/UX designs, enriches user experience, and helps us express ourselves fully to our users.
You might be now concerned that it would quite the hassle to add customised pagination in your app. Let us just begin this segment by saying, things on ReactJS are always being built to make the lives of developers simpler. The React 16.8 version introduced hooks which are functions that essentially work by “hooking onto” the state or lifecycle features from function components in React. This means we can write functions (or hooks!) using React features and states without having to write a class. TLDR; we can create pagination hooks of our own in ReactJS and make the task of adding custom pagination to our app rather simple.

A custom pagination hook in ReactJS can be created using five simple steps:
- Setting up the project
- Defining props for pagination interface
- Determining the customisations for the pagination hook
- Building the custom pagination hook
- Implementing pagination component
Let us now dive into these steps individually.
Setting Up the Project
First, we use the create-react-app command line package to set up a new React Project for our custom pagination hook. This package can be globally installed by using npm install -g create-react-app or yarn add global create-react-app.
Now start a new project we run create-react-app from the command line:
Now we must install the dependencies for our hook. Here I am only using one simple additional dependency which is classnames. This would allow me flexibility while handling different classNames with different conditions.
We can install this by using yarn add classnames and run the project with the command:

Defining Props for Custom Pagination Component
Now we can move on to discussing the values we would be needing props to incorporate into our pagination hook.
- totalCount: this value indicates the total count of data present in the source.
- currentPage: this indicates the current active page. For the sake of a customisation example, let us implement the currentPage value of a 1-based index for our hook instead of the commonly used 0-based index.
- onPageChange: This is a callback function which is invoked when a page is changed.
- pageSize: this value decides the maximum data that would be visible per page.
- siblingCount: This value tells the minimum number of page buttons that would be shown on the two sides of the current page button. This is an optional prop with the default value of 1.
- className: This too is an optional prop indicating the className that can be added to a top-level container.
Determining the Customisations for Our Pagination Hook
For this project, let us create a very simple custom pagination hook. We are going to make our customised hook to be able to implement a chosen range of numbers on the pagination component of the apps where it is called, and this chosen range of numbers is going to be joined by dots when the final range is returned. Our pagination hook will depend on totalCount, currentPage, pageSize, and siblingCount for computing the page ranges.
We are calling our pagination hook usePagination. Please note that use works as a constructor by defining initial values required by the functional component. Any hook with the prefix use is a custom ReactJS Hook that can call other Hooks.
There are a few things to consider before beginning to determine the code skeleton of our usePagination hook. Firstly, we are going to ensure that the displayed range of numbers in our pagination component must be returned by our hook in the form of an array.
Secondly, our computation logic should re-run every time the values of our props totalCount, currentPage, pageSize, or siblingCount are changed. For this project, we will invoke the useMemo hook for the computation of our core logic and it will re-run when the values of props are altered.
Lastly, and most importantly, the total number of items that our hook returns must remain constant to avoid issues like resizing of the pagination component when range array length changes during user interaction.
With the above considerations in mind, we are finally going to start building our usePagination hook by creating a file named usePagination.js in the src folder of our new pagination project. Check out the skeleton code for our custom pagination hook:
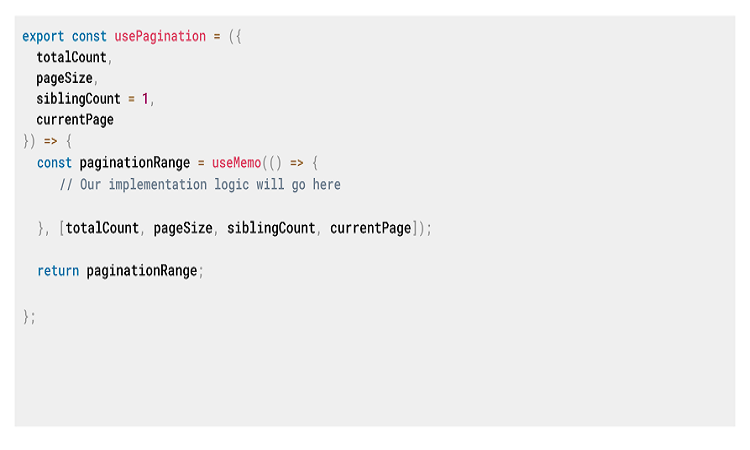
Note how we have set the defaultValue of siblingCount as 1, just like we discussed in the previous segment.
Building our Custom usePagination Hook
First, we need to compute the total number of pages from totalCount and pageSize. Here we will use Math.ceil which will round off the page number to its immediate higher integer value, ensuring that an extra page is reserved for the remaining data.

Now we can proceed to give our hook a custom range function. This range function would take in specified start and end values to return an array consisting of elements from start to end.
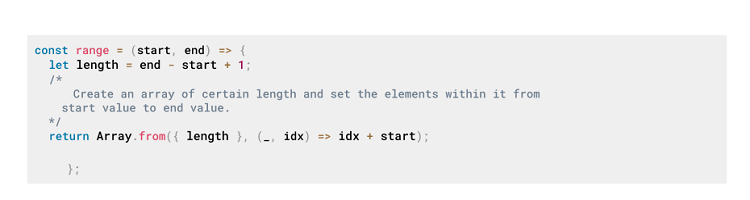
At this point, our custom usePagination hook is almost built. All we need to do now is implement our core logic.
Let us consider the possible scenarios before coding the core logic. In the first scenario, consider that the value of totalPageCount is lesser than the number of navigation components (pills) we are getting depending on our chosen props. In this case, we would only return a range of numbers 1.
totalPageCount
In the other scenario, the value of totalPageCount exceeds the number of pills. In this case, we would have to decide if our pagination bar needs dots to the left or right of the currentPage. We can do this by simply calculating the respective indices after we include the siblingCount to the currentPage. Now that we have determined how the dots would be placed on the pagination bar, we can finally finish the code.


Our custom usePagination is now all built!
Our pagination component will be rendered as a pagination tab with left and right arrows. We map the paginationRange and render page numbers as pagination-items, and if the total number of pages is less than two, we are opting for not having a pagination bar and return null.
Here’s the CSS file should look:


We are all done! We just discussed how to add pagination to our apps in ReactJS and even built a standard custom Pagination hook. Now we can delve into our imaginations to build more complex custom Pagination hooks in ReactJS and add that extra subtle detail to our apps!